If you’ve learned any programming (in just about any language) you’ve learned about For Loops:
For as long as (i is less than (the point I want you to stop at) ) do something.
It’s how you do something X times. (Really, i times, but you get me. 😉)
Like print out your name 1,000 times so it fills the screen.
Really common in programming and pretty easy. And perhaps not that useful, but it definitely has its moments.
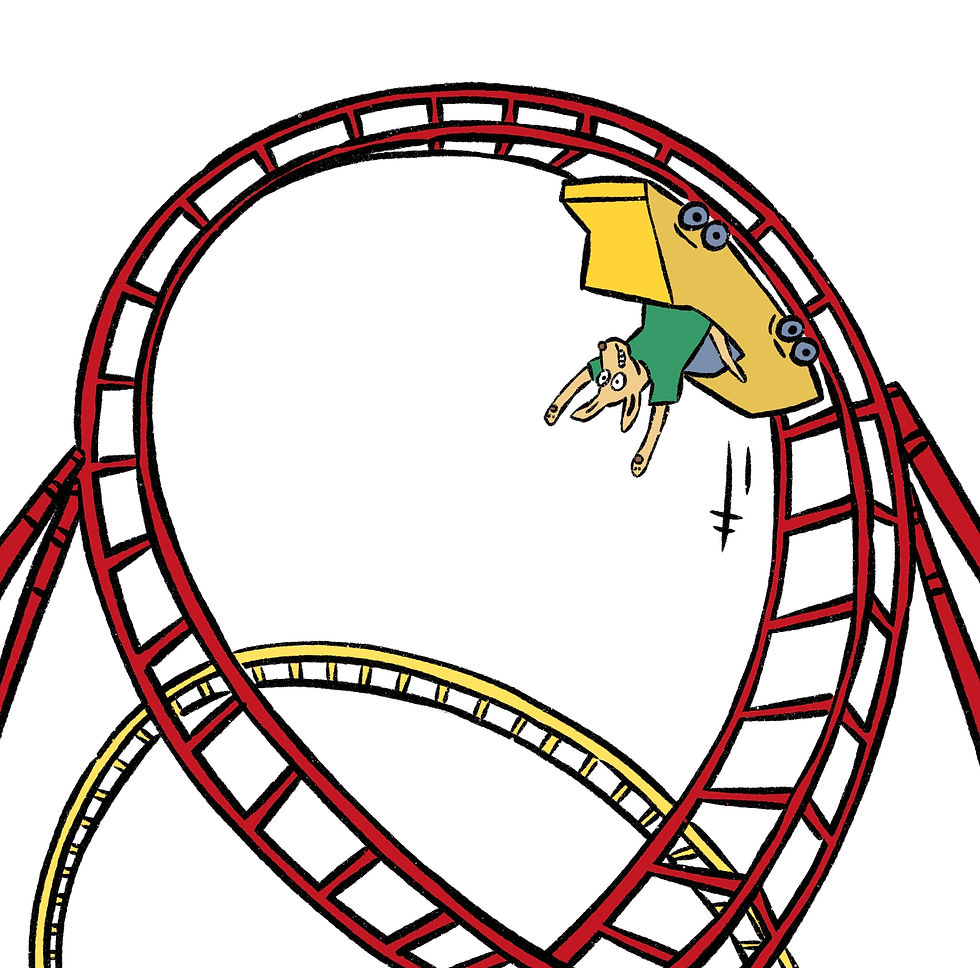
But guess what’s not straightforward in Flow?
Flow loves to do loops over collection variables, where you already have the records you’re going to work through and do something about or to each of them. Most of the time that’s what you’d want to do in a flow, sure.
But what if you want to do something a particular number of times? Super easy in programming, not straightforward in Flow.
There’s no simple Flow equivalent of “i++”, the syntax for incrementing a counter to control your For Loop. If you make a flow collection variable that holds numbers, which you could technically loop over, you would have to add each number to that collection. The work of doing that would be more effort than it's worth.
Maybe when you get a new volunteer you want to automatically set up ten check-ins with them to see how their volunteer journey is progressing?
Or a table sponsorship entitles the purchasor to 11 additional guests at the gala, for a whole 12 seat round table.
There are all sorts of reasons you might need to either do something X times or create X of something.
Let’s look at how you can actually accomplish this in flow.
We’re going to look at an example that does something ten times. You could easily replace ten with any other number.
1. The first thing we need is our counter. That’s going to be a number variable that we’re going to use to increment. I’ve called mine “i” for the purposes of this blog.
But I will note that my normal naming convention for variables in flow is to start them with “var.” So in real life I would call this something like “varCounter” or “varIterationCounter.” But in programming a For Loop we would use just i, so that’s how I’m showing it here.
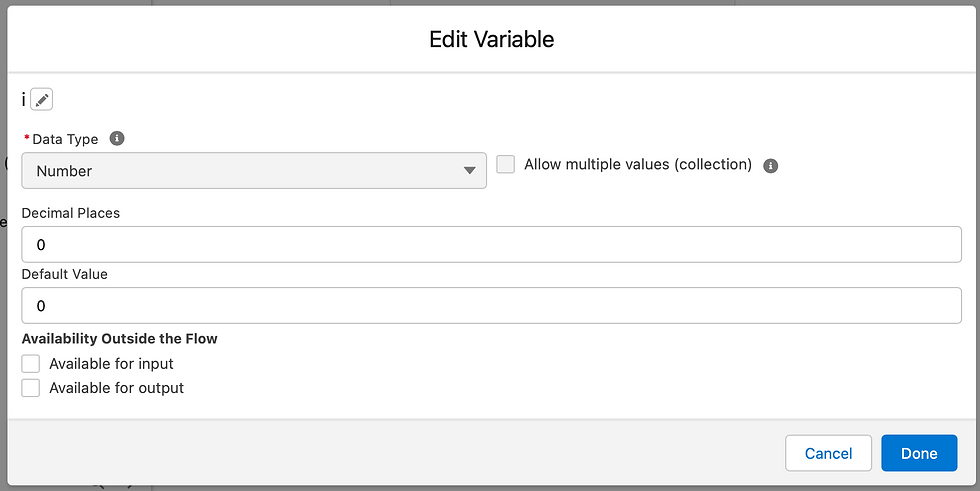
2. The first actual step in the flow (or this loop portion of the flow) is a Decision. You have to check if your counter has reached the stopping point. We want to see if i < 10 or if it has reached 10 or more.
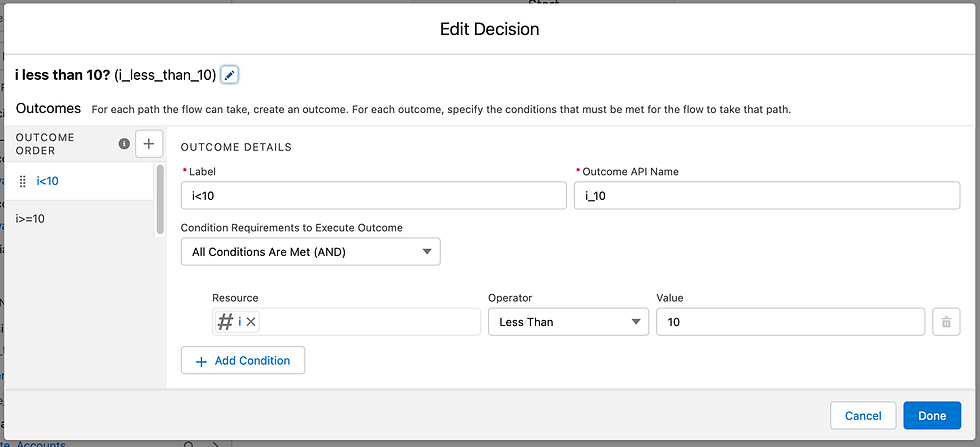
Note: I started my counter, above, at zero, so I have to stop one lower than my desired number of iterations.
Computers like to count "0, 1, 2, 3, …" If you reach 10, you’ve done something eleven times. If this confuses you, you could start your counter at 1 instead of zero (set the default above to 1, rather than 0.) You also could start a counter at the top and work your way down. That would be i-- rather than i++, which is totally acceptable though less commonly used.
(Also, by the way, word processors really want to autocorrect “i minus minus” into “i em-dash.”)
3. If we are still in the loop (our counter has not reached 10), then we
“do something” and
iterate our counter.
(This would be the code block within the For Loop.)
The “do something” part of your flow, of course, depends on what you need to do. For our example we are making a flow that will create 10 accounts, so we have to assign a record variable for one of those accounts.
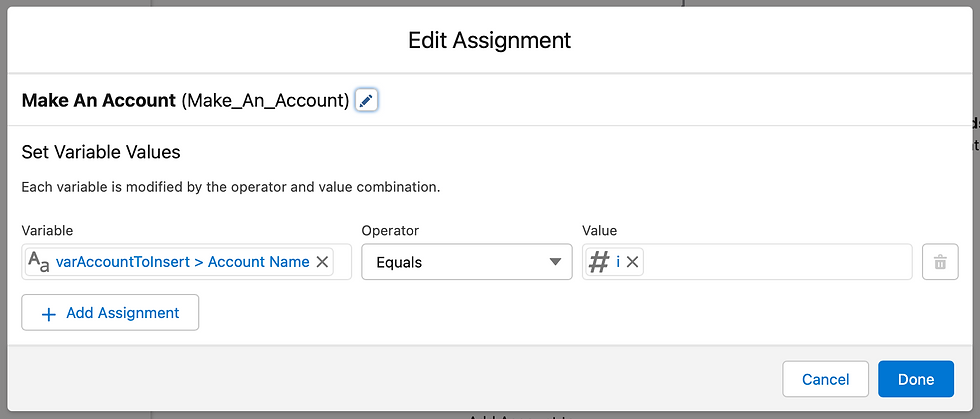
To iterate the counter you are going to use an Assignment element to add 1 to the variable i.

(I know I said that Flow doesn't do i++, but that's exactly what this step is doing. It just doesn't read that way.)
4. Then we have to add the account record variable we just assigned to a collection variable (for insertion later.)

You can actually use a single Assignment step to both add the record variable to the collection and to increment the counter. But I wanted to show the entire idea diagrammed out. Also: You can not assign your record variable's fields and assign that record variable to the record collection variable in the same step. That will not work.
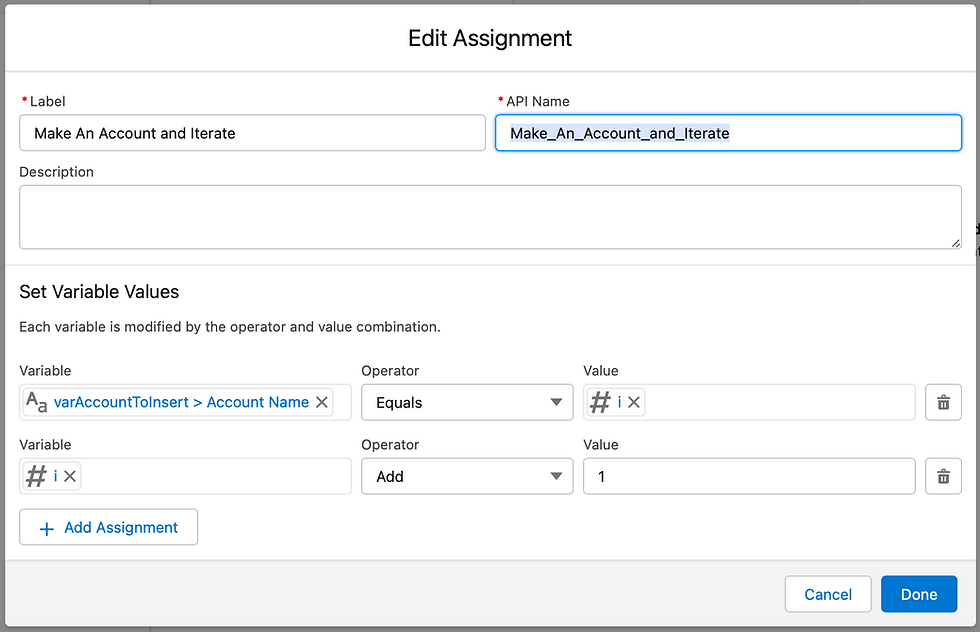
5. We loop back to the decision element to see if we need to do this again.
6. If we have reached the limit, then we break out of our loop and use the Create Records element to actually put 10 new accounts into the database.
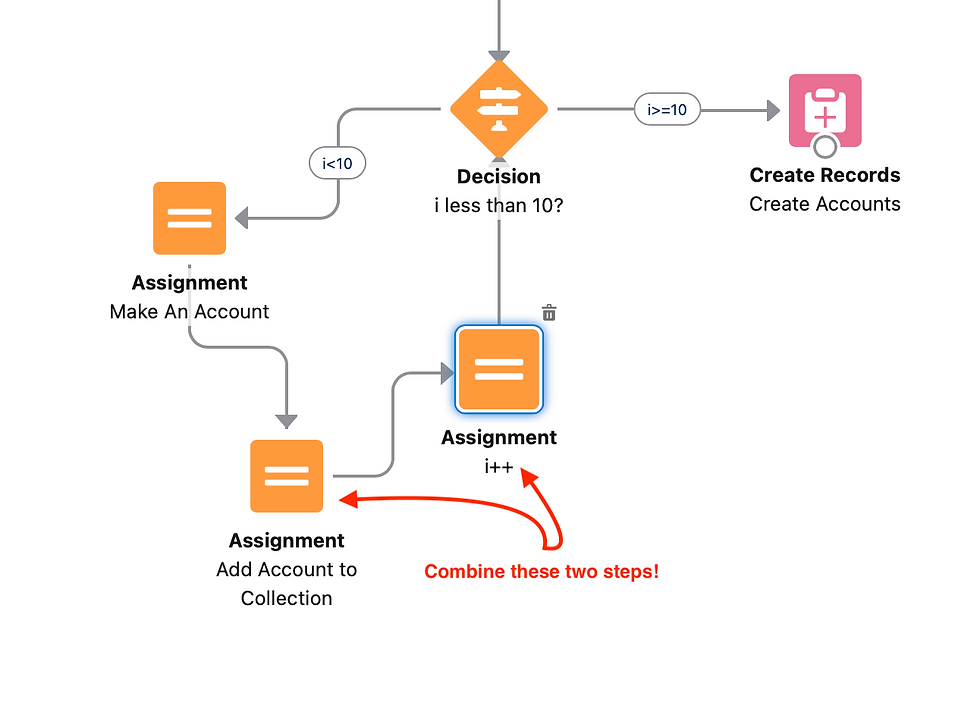
Et voila!
Here are all the accounts that were in our collection variable now available in the database.
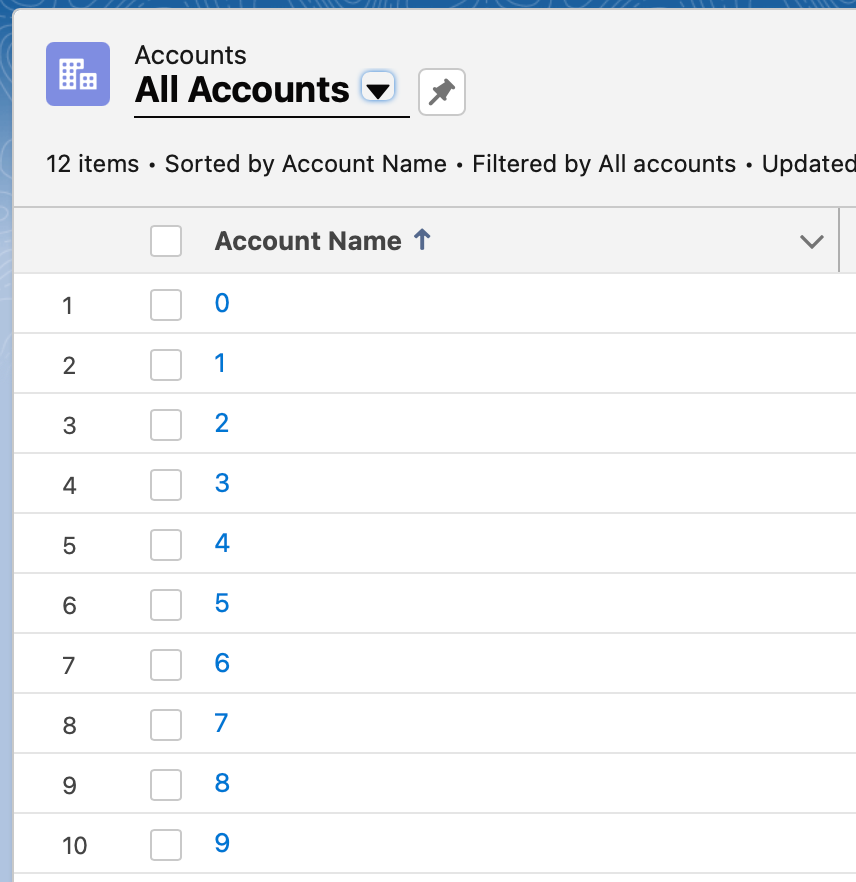
Summing it all up, here’s the most simple representation of what we’re doing, though for it to work in Flow you need at least one additional assignment element, as I noted above.
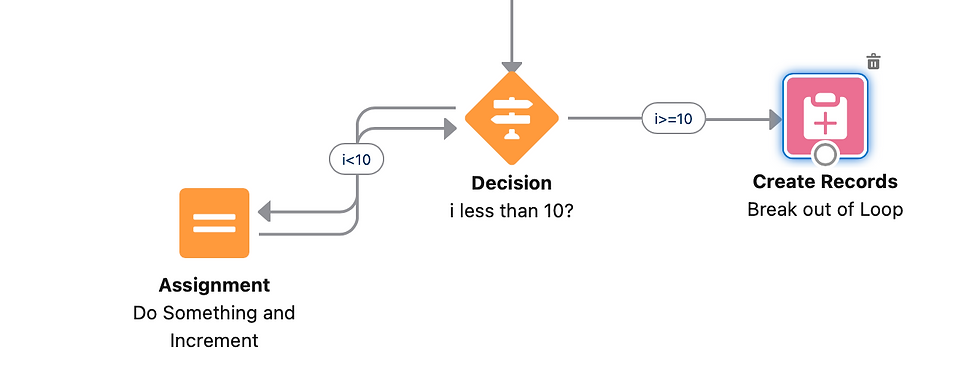
Once you know how to do it, this is really not such a big deal! But if you're new to Flow or haven't needed to solve this particular challenge before it takes a moment to puzzle it out.